Table of Contents
Introduction To Visitor Design Pattern
Visitor design pattern is a tool in object-oriented programming for introducing new operations without altering existing class structures. It simplifies navigation through complex object networks by segregating operations from the elements they affect.
When an Element object accepts a Visitor object, double dispatch is employed, meaning the appropriate method on the Visitor is invoked based on both Visitor and Element types.
For instance, in a zoo scenario, the Visitor pattern could manage various interactions, such as feeding and grooming animals.
The pattern’s value lies in its ability to maintain a clean codebase while allowing for the addition of new operations without disrupting existing code. Each operation is encapsulated within its Visitor class, promoting modularity and code organization.
This clarity facilitates easier navigation through the codebase, enhancing readability and understanding for developers. In summary, the Visitor pattern offers simplicity, clarity, and adaptability in managing complex object structures.
How can you determine when to apply the Visitor design pattern to address a problem?
Identifying the need for the Visitor design pattern involves recognizing certain patterns or scenarios within your problem statement.
- Diverse Operations on a Complex Object Structure:
- When you have a complex object structure with multiple types of elements and each element requires different operations to be performed on it.
- Avoiding Modification of Existing Classes:
- If you want to introduce new operations to existing classes without altering their structure or violating the open/closed principle.
- Adding New Operations Frequently:
- When you anticipate the need to add new operations frequently, and you want to ensure that the addition of these operations doesn’t lead to code clutter or tight coupling.
- Double Dispatch Requirement:
- When you have scenarios where the appropriate method to be invoked depends on both the type of the element being operated on and the type of the operation being performed.
Example: Document Processing System using Visitor Design Pattern
Imagine you’re developing a document processing system where you need to perform various operations on different types of elements within documents, such as text, images, and tables. Each element may require different operations to be performed on it. For instance:
- Text elements might need operations like formatting, spell checking, and word count.
- Image elements might need operations like resizing, cropping, and applying filters.
- Table elements might need operations like sorting, filtering, and calculating totals.
Without the Visitor pattern, you might be tempted to add these operations directly to the classes representing each type of element. However, this could lead to a bloated and tightly coupled design, making it difficult to add new operations in the future without modifying existing classes.
Instead, by employing the Visitor pattern, you can define separate Visitor classes for each operation. Each Visitor class encapsulates the logic for a specific operation. The elements accept Visitor objects, which then execute the appropriate operation based on the type of element being visited.
For example, you could have a FormatVisitor class for formatting text elements, an ResizeVisitor class for resizing image elements, and a CalculateVisitor class for calculating totals in table elements. Each Visitor class knows how to perform its designated operation on the respective element type.
This approach allows you to add new operations to the system by simply creating a new Visitor class, without modifying the existing classes representing document elements. It promotes modularity, extensibility, and maintainability in your document processing system.
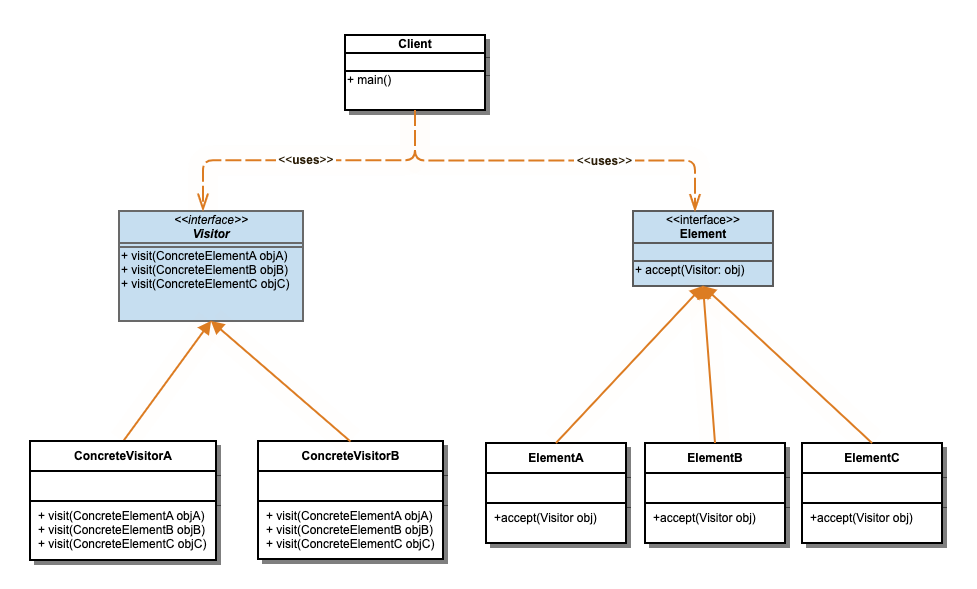
Sample Code for Visitor Design Pattern
// element interface
public interface HotelRoom {
public void accept(RoomVisitor visitor);
}
// Concrete element class
public class SingleRoom implements HotelRoom {
public int roomPrice = 0;
@Override
public void accept(RoomVisitor visitor) {
visitor.visit(this); //double dispatch
}
}
// Concrete element class
public class DoubleRoom implements HotelRoom{
public int roomPrice = 0;
@Override
public void accept(RoomVisitor visitor) {
visitor.visit(this); //double dispatch
}
}
// Concrete element class
public class DeluxeRoom implements HotelRoom{
public int roomPrice = 0;
@Override
public void accept(RoomVisitor visitor) {
visitor.visit(this); //double dispatch
}
}
//visitor interface
public interface RoomVisitor {
public void visit(SingleRoom singleRoomObj);
public void visit(DoubleRoom doubleRoomObj);
public void visit(DeluxeRoom deluxeRoomObj);
}
//Concrete visitor class
public class RoomPricingVisitor implements RoomVisitor{
@Override
public void visit(SingleRoom singleRoomObj) {
System.out.println("Pricing Computation Logic of SingleRoom..!");
singleRoomObj.roomPrice = 1000;
}
@Override
public void visit(DoubleRoom doubleRoomObj) {
System.out.println("Pricing Computation Logic of DoubleRoom..!");
doubleRoomObj.roomPrice = 2000;
}
@Override
public void visit(DeluxeRoom deluxeRoomObj) {
System.out.println("Pricing Computation Logic of DeluxeRoom..!");
deluxeRoomObj.roomPrice = 5000;
}
}
//Concrete visitor class
public class RoomMaintenanceVisitor implements RoomVisitor{
@Override
public void visit(SingleRoom singleRoomObj) {
System.out.println("Performing maintenance of Single Room..!");
}
@Override
public void visit(DoubleRoom doubleRoomObj) {
System.out.println("Performing maintenance of Double Room..!");
}
@Override
public void visit(DeluxeRoom deluxeRoomObj) {
System.out.println("Performing maintenance of Deluxe Room..!");
}
}
public class Client {
public static void main(String[] args) {
SingleRoom singleRoomObj = new SingleRoom();
DoubleRoom doubleRoomObj = new DoubleRoom();
DeluxeRoom deluxeRoomObj = new DeluxeRoom();
RoomVisitor pricingVisitorObj = new RoomPricingVisitor();
singleRoomObj.accept(pricingVisitorObj);
System.out.println(singleRoomObj.roomPrice);
doubleRoomObj.accept(pricingVisitorObj);
System.out.println(doubleRoomObj.roomPrice);
deluxeRoomObj.accept(pricingVisitorObj);
System.out.println(deluxeRoomObj.roomPrice);
RoomVisitor maintenanceVisitorObj = new RoomMaintenanceVisitor();
singleRoomObj.accept(maintenanceVisitorObj);
doubleRoomObj.accept(maintenanceVisitorObj);
deluxeRoomObj.accept(maintenanceVisitorObj);
}
}