Composite design pattern is a structural pattern that allows you to compose objects into tree-like structures to represent part-whole hierarchies.
Composite design pattern is like organizing things in folders on your computer. Just as a folder can contain files or other folders. This allows you to treat individual objects and groups of objects in a unified way. So, whether you’re working with a single object or a whole collection, you can use the same operations and methods to interact with them.
5 real world example of Composite design pattern
File System: Folders in a file system can contain files or other folders, demonstrating the Composite pattern’s hierarchy.
Menu in Software Applications: Software applications often have menus with submenus, allowing users to navigate through various options and functionalities in a structured manner.
Educational Courses: Online learning platforms organize educational courses hierarchically, with each course containing modules, lessons, quizzes, and assignments.
Organization Chart: An organization chart can represent a hierarchical structure of employees and departments, mirroring the Composite pattern.
Playlist Management: A music player application can organize playlists hierarchically, with each playlist containing individual songs or sub-playlists.
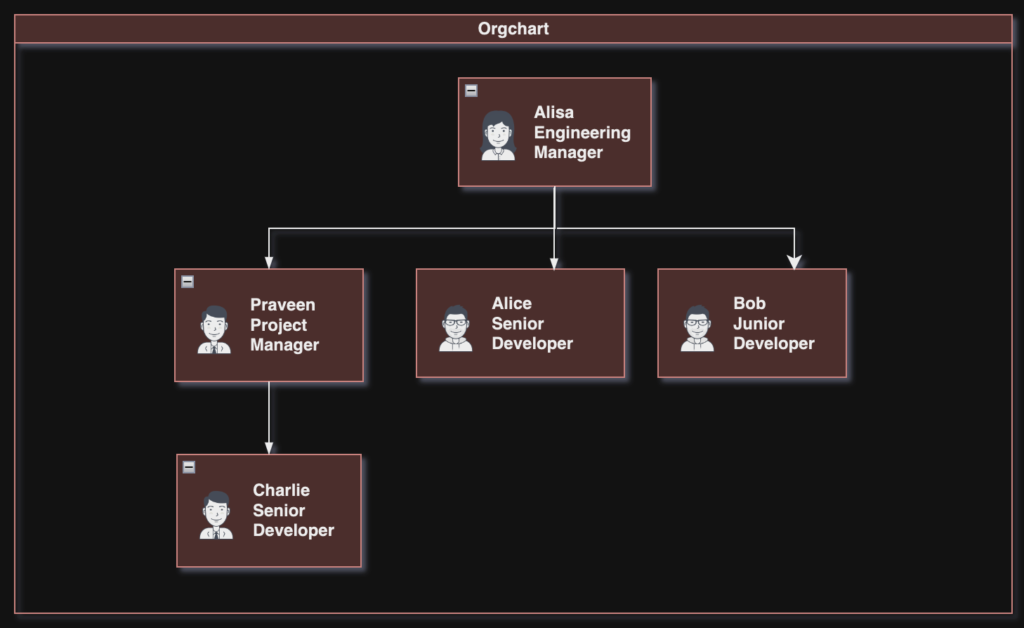
~:Sample code for Organizational structure using Composite Design Pattern:~
// Component interface
interface Employee {
void showDetails();
}
// Leaf class representing individual employees
class Developer implements Employee {
private String name;
private String position;
public Developer(String name, String position) {
this.name = name;
this.position = position;
}
@Override
public void showDetails() {
System.out.println("Developer: " + name + ", Position: " + position);
}
}
// Composite class representing Manager or teams
class Manager implements Employee {
private String name;
private List<Employee> subordinates = new ArrayList<>();
public Manager(String name) {
this.name = name;
}
public void addSubordinate(Employee employee) {
subordinates.add(employee);
}
@Override
public void showDetails() {
System.out.println("Manager: " + name);
System.out.println("Subordinates:");
for (Employee employee : subordinates) {
employee.showDetails();
}
}
}
public class Main {
public static void main(String[] args) {
// Create leaf employees
Employee developer1 = new Developer("Alice", "Senior Developer");
Employee developer2 = new Developer("Bob", "Junior Developer");
Employee developer3 = new Developer("Charlie", "Senior Developer");
// Create composite managers
Manager engineeringManager = new Manager("Engineering Manager");
Manager projectManager = new Manager("Project Manager");
// Add employees to managers
engineeringManager.addSubordinate(developer1);
engineeringManager.addSubordinate(developer2);
projectManager.addSubordinate(developer3);
// Add project manager to engineering manager
engineeringManager.addSubordinate(projectManager);
// Show details of engineering manager (including subordinates)
engineeringManager.showDetails();
}
}